3.6 - Color¶
The most basic surface property of a model face is its color.
Color Models¶
For devices that produce images using light, such as computer monitors, color is modeled as a combination of three base colors: red, green and blue. Light is additive. If you add red, green and blue light at full intensity, you get white light. The absence of light produces black. All colors discernible by the human eye can be created using some combination of red, green and blue light. This is called the RGB (red, green blue) color system.
For devices that produce images from reflected light, such as printed pages, color is modeled as a combination of three base colors: cyan, magenta and yellow. These pigments absorb certain wavelengths of light and basically reflect red, green and blue light. So this is not really a different way to represent color, it is just a different way to produce reflected light. All colors discernible by the human eye on a printed page can be created using some combination of cyan, magenta and yellow pigments. This is called the CMY (cyan, magenta, yellow) color system. Printers typically also use blank pigment to get crisper black colors. This is called the CMYK system, where K stand for “blacK”.
Colors represented in RGB can be converted to CMYK, and vis versa. We will concentrate on RGB colors because that is what WebGL programs typically use.
We need three values to represent a specific color: how much red, green, and blue light. It makes sense to make these values percentages: 0% means no color, while 100% means full color. It is up to the hardware of a device to determine what 100% of a color means. You will quickly recognize this if you looked at various brands of TV’s in a store. They don’t all recreate the same colors from the same data!
Device Independent Color¶
Remember that one of the goals of creating WebGL programs in the browser is that the graphics will execute correctly in cross-platform environments. We could like to specify colors that are device independent. The standard scheme for device independent colors uses floating point values. Therefore, (0.45, 0.23, 0.89) represents a color that has 45% red, 23% green, and 89% blue.
How many unique colors can be represented using floating point values? A lot! Each component value can represent approximately 8 million different percentage values. This allows for approximately 600,000,000,000,000,000,000 possible colors! Can the human eye see that many colors? The answer is no! The typical person can only see about 250 different variations in any particular shade of color, though color perception does vary from person to person. Consider the image below that displays various shades of black and white for various color depth choices.
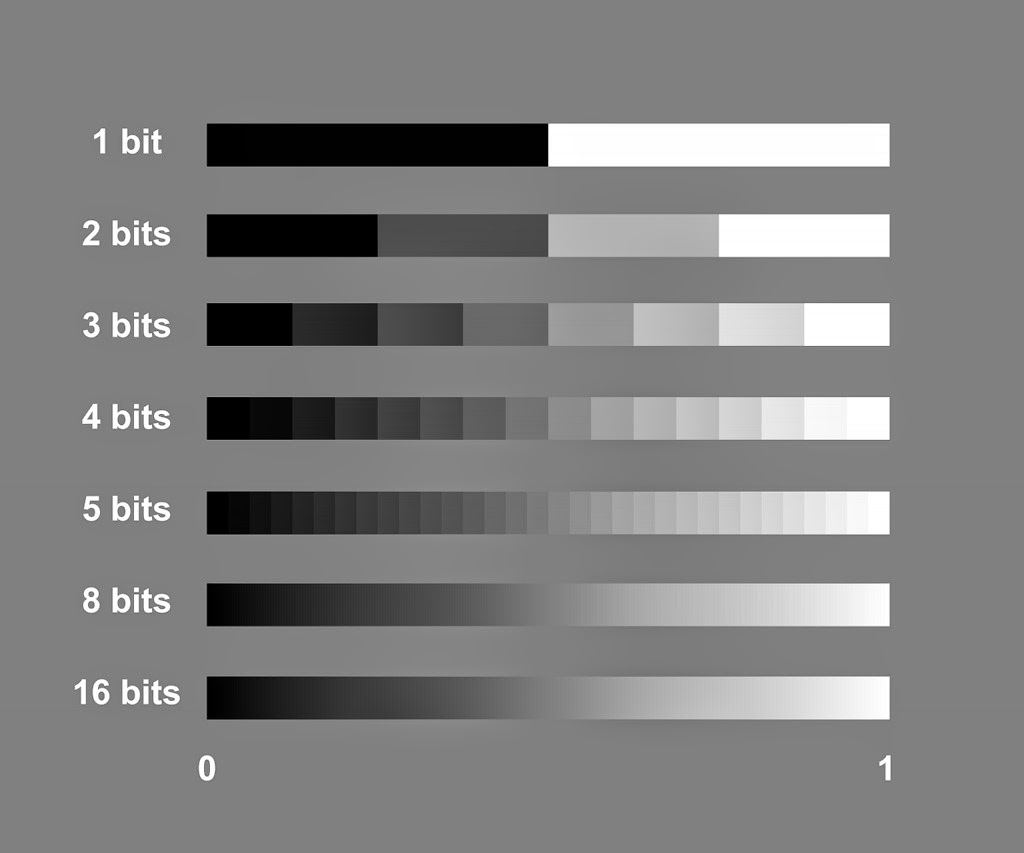
Color depth for shades of black and white. (1)
Notice the following facts about the image above:
- Each block of color is one solid color, even though it looks like the color is changing within a block. This phenomenon is called mach bands. To prove to yourself that each block of color above is one solid color, take two pieces of paper and cover up the block of color on either side of a specific block. The block will become one uniform color! Remove the coverings and the “banding” effect will reappear. Your eye is designed to enhance contrast!
- For most people, the 8 bit color depth provides a smooth progression of color. Can you see any mach bands in the ‘8 bits’ image? If not, this is at or above your eye’s maximum color resolution.
- The ‘8 bits’ color depth allows for 256 different shades of black and white. If you use 8 bits for each RGB component value, you can represent over 16 million different colors. (2 8) 3. For the average person this color precision is considered “full color”.
Transparency¶
An opaque object looks solid and reflects basically all of the light that strikes it. A transparent object allows some light to pass through it. The amount of transparency of an object can vary widely based on its composition. For example, a glass cup might be highly transparent, while water is partially transparent. Accurately modeling transparency is very difficult. A very simplistic model for transparency is to store a “percentage of opaqueness” with a color value. This percentage is called the “alpha value”. The RGBA color system stores 4 component values for a color, where the first three values are the red, green and blue components and the last value is the alpha value. You will also see references to ABGR and ARGB color representations. The order of the letters indicates the order of the values in the color specification.
Using floating point percentages for each component, here are a few examples:
RGBA color | Opaque | Transparent |
---|---|---|
(0.2, 0.1, 0.9, 1.0) | opaque, all light is reflected | 0% transparent |
(0.2, 0.1, 0.9, 0.9) | mostly opaque | 10% transparent |
(0.2, 0.1, 0.9, 0.5) | half the light passed through the object | 50% transparent |
(0.2, 0.1, 0.9, 0.0) | all light travels through the the object, the object is invisible | 100% transparent |
Shades of Color¶
Notice that since (0,0,0) is black and (1,1,1) is white, shades of any particular color are created by moving closer to black or to white. You can use the parametric equation for a linear relationship between two values to make shades of a color darker or lighter.
A parametric equation to calculate a linear change between values A and B:
C = A + (B-A)*t; // where t varies between 0 and 1
To change a color (r,g,b) to make it lighter, move it closer to (1,1,1).
newR = r + (1-r)*t; // where t varies between 0 and 1
newG = g + (1-g)*t; // where t varies between 0 and 1
newB = b + (1-b)*t; // where t varies between 0 and 1
To change a color (r,g,b) to make it darker, move it closer to (0,0,0).
newR = r + (0-r)*t; // where t varies between 0 and 1
newG = g + (0-g)*t; // where t varies between 0 and 1
newB = b + (0-b)*t; // where t varies between 0 and 1
// or
newR = r*t; // where t varies between 1 and 0
newG = g*t; // where t varies between 1 and 0
newB = b*t; // where t varies between 1 and 0
WebGL Color¶
All colors in WebGL require a four-component, RGBA, value. If you don’t specify an alpha value, it is assumed to be 1.0 (opaque). WebGL 1.0 requires that all vertex attributes be floating point values. Therefore, opaque color values will be stored as 3 floating point values, while color values that allow for transparency will require 4 floating point values.
Glossary¶
- color model
- A mathematical representation for color.
- RGB color model
- Represent a color for a light emitting device using 3 percentage values, one for red, green, and blue light.
- CMY color model
- Represent a color for a printed page using 3 percentage values, one for cyan, magenta, and yellow pigment.
- CMYK color model
- Represent a color for a printed page using 4 percentage values, one for cyan, magenta, yellow and black pigment.
- color depth (or bit depth)
- The number of bits (binary digits) used to represent a color component value.
- full color
- Use a color depth large enough to represent the full range of color the average person can see. This is typically recognized to be 8 bits per component; 24 bits for RGB colors; 32 bits for RGBA colors.
- opaque
- An object that reflects all of the light that hits it; not transparent.
- transparent
- An object’s composition allows some of the light that strikes its surface to pass through. A person sees both the transparent object and the object behind.
- alpha component
- The percentage of light that is reflected from an object. (1.0 - alpha) is the percentage of light that passes through an object.