3.8 - Light Modeling¶
To model how an object looks in the real world you have to model how light interacts with the surfaces of an object. One or more of the following four things happens when light strikes an object:
- The light reflects and goes off in a different direction. The direction of the reflected light is determined by the surface properties of the face.
- The light is absorbed by the object and converted to energy, which heats up the object over time. (This is called absorption.)
- The light travels through the object and continues on, but at a different trajectory. (Light traveling through an object is called transparency and the change in direction is called refraction.)
- The light enters the object, bounces around inside the object, and then leaves the object at a different place than it hit. (This is called subsurface scattering.)
Modeling all of these interactions is complex and very difficult. It can be done, but it is beyond the scope of these basic tutorials. We will only discuss how light reflects off of an object. You can’t produce photo-realistic images using just reflected light, but you need to understand the basics before attempting more complex modeling.
Light Sources¶
In the real world light comes from light sources. The sun is the most obvious light source. Other light sources include lamps, spot lights, fire and explosions. The characteristics of light changes based on its source. We need to model some of these basic properties of light if we hope to get reasonable renderings. We will model the following properties of a light source:
- Position - where is the light coming from? There are two scenarios:
- Directional - The light source is so far away that all light rays are basically traveling in the same direction. The sun is a directional light source.
- Positional - The light source is inside the scene. The angle that light strikes an object changes based on their relative position. Note that the angle the light strikes individual vertices of an individual triangle will be different.
- Color - for example, a red spot light.
- Point vs Spotlight - does the light travel in all directions, or is the light restricted to a specific direction, such as flashlight.
Since light can have different colors, we model light in the same way we model surface color using a RGB value. A red light would be (1.0, 0.0, 0.0) while a white light would be (1.0, 1.0, 1.0).
Ambient Reflected Light¶
Sometimes you don’t know the source of the light in a scene. For example, think about being in a dark room. You can see things, but you don’t necessarily know where the light in the room is coming from. It might be coming from the moon through a window, or from a light in another room under a doorway, or the faint glow of a night light. Light that is not coming directly from a light source, but is just bouncing around in a scene, is called ambient light.
Ambient light is “background” light. It bounces everywhere in all directions and has no specific location of origin. Ambient light illuminates every face of a model. Therefore, both the faces that get direct light, and the faces hidden from direct light are illuminated with the same amount of ambient light.
The amount of ambient light determines the overall light in a scene. An ambient light of (0.1, 0.1, 0.1) would model a dark room, while an ambient light of (0.4, 0.4, 0.4) would model a well lit room. An ambient light of (0.2, 0.0, 0.0) would simulate a low intensity red light permeating a scene. The exact values you use will typically be based more on experimental results than on actual physical properties of a scene.
Diffuse Reflected Light¶
The amount of light that is reflected off of the surface of an object is related to the orientation of the surface to the light source. If light hits the surface “straight on”, most of the light will be reflected. If light just “grazes off” the side of an object, then very little light is reflected. This is illustrated in the diagram.
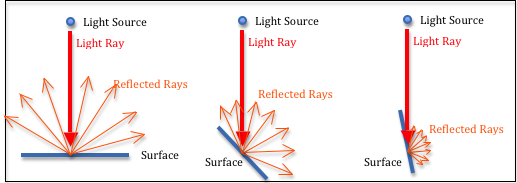
Diffuse light reflection.
We assume that the surface of a face is not perfectly smooth and that the light scatters equally in all directions when it reflects off the surface. For diffuse light the question is how much reflection occurs, not the direction of the reflection. In Physics, Lambert’s cosine law gives us the amount of reflection.
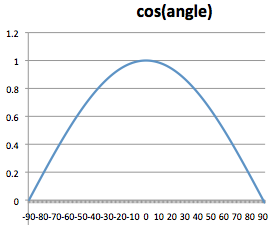
If we take the cosine of the angle between the surface normal vector and the light ray, this gives us the amount of reflected light. When the angle is zero, cos(0) is 1.0 and all the light is reflected. When the angle is 90 degrees, cos(90) is 0.0 and no light is reflected. If the cos() is negative, the face is orientated away from the light source and no light is reflected. The amount of reflected light is not a linear relationship, as you can see in the plot of the cosine function in the image.
If you multiply the color of a surface by the cosine of the angle between the surface normal and light ray, you will scale the color towards black. This is exactly the results we want. As less and less light reflects off of a surface, the surface becomes darker.
Specular Reflected Light¶
If an object is smooth, some of the light reflected off of the surface of an object can be reflected directly into the viewer’s eye (or the camera’s lens). This creates a “specular highlight” that is the color of the light source, not the color of the object, because you are actually seeing the light source light. Each of the white areas on the blue balls in the image is a specular highlight.
The location of a specular highlight is determined by the angle between a ray from the viewer to the point on the surface, and the exact reflection of the light ray. The surface normal is used to calculate the reflected light ray. Please study the diagram below.
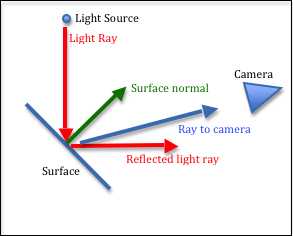
Specular highlight (Angle between reflected ray and ray to camera.)
WebGL Implementation¶
All lighting effects in WebGL are done by the programmer in a fragment shader. To implement the three lighting effect described above, you would do the following:
- Get the amount of ambient light from a light source model.
- Calculate the angle between the surface normal vector and the light direction.
- Multiply the cosine of the angle times the surface’s diffuse color.
- Calculate the angle between the light reflection and the camera direction.
- Multiply the cosine of the angle times the light model’s specular color.
- Add the ambient, diffuse, and specular colors. This is the color of the pixel for this fragment of the triangle’s surface.
We will cover the details of implementing a lighting model in Sections 10 & 11.
Glossary¶
- light model
- A mathematical description of a light source.
- ambient light
- Light in a scene that has no discernible source. All faces of all models are illuminated with ambient light.
- diffuse light
- Light that directly strikes an object and then reflects in all directions. The amount of reflection is determined by the angle between the light ray and the surface normal vector.
- specular light
- Light that reflects off of a smooth surface directly into the lens of a camera. The color of the light, not the color of the surface, is rendered.