1.5 - Software Organization For WebGL programs¶
A WebGL program is made up of a significant amount of software. Without good software organization and abstraction, your ability to maintain and enhance these graphic programs over time will be greatly diminished. Most programmers hate having a prescribed software structure imposed on them. There are many ways to design software and a good design by one programmer might be confusing to a different programmer. But there is no getting around the fact that a well designed software structure is needed.
JavaScript supports object-oriented programming and the software in these tutorials is designed as a collection of objects for the following very important reasons.
- Javascript has a large number of variables and functions in its global address space. You should always design software that limits the number of values and functions that are defined globally, but even more so for JavaScript. This protects you from overwriting and redefining built-in Javascript functionality.
- Good software design mandates that data and its related functionality be combined into a logical unit. This creates a logical organization to your code, and protects the data inside each object from accidental modification.
- Your code should be structured in a way that facilitates multiple 3D graphics renderings in multiple canvas windows on a single web page. Creating Javascript classes that can be used to create separate object instances, one for each canvas rendering, just makes sense.
- Some of your Javascript code can be implemented generic enough to be re-used for a variety of graphics projects. Other code will be very program specific. If you don’t separate generic code from program specific code, you will limit your code re-use. WebGL applications are complex and you will want to re-use code as often as possible.
- WebGL code is very interdependent. If you change one statement in a shader program it might require you to change many aspects of your related Javascript code. It will help you to separate interdependent code from other “standalone” code.
- The importance of isolating one-time initialization code from repeatedly executed rendering code can’t be overstated. Your code should make it very clear which logic is executed only once as a preprocessing step. This will make it easier for you to optimize your graphics for real-time rendering speeds.
A sample of the software structure used in these tutorials is shown in the UML (Unified Modeling Language) diagram below. The arrows indicate relationships. The classes in blue boxes are static Javascript libraries that remain unchanged from one WebGL program to another. The classes in the orange boxes are Javascript code that changes based on the sophistication and type of graphics being produced.
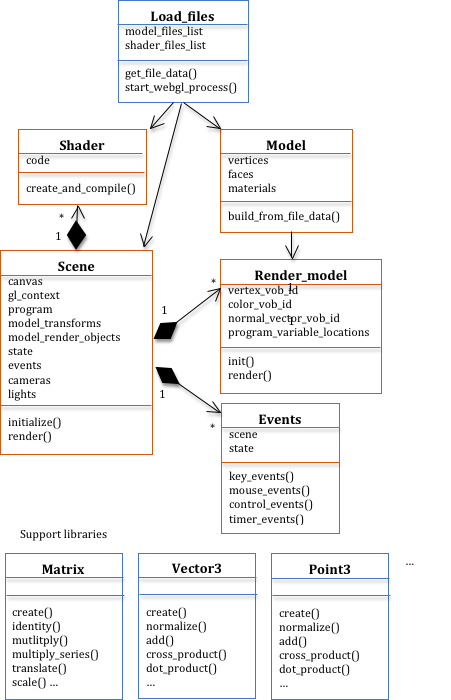
The details of the UML diagram above are not important at this point. What is important is that you understand that object-oriented programming will be critical to the successful implementation of your WebGL programs. For example, a scene is typically composed of multiple models. It makes sense to create an object for each model. This isolates the complexity of each model in a separate JavaScript object and it greatly facilitates code re-use.
The Javascript classes defined in this software design are written with understanding and clarity in mind. They are well documented, broken into separate files, and coded using descriptive names. These classes are open-source and intended for your re-use. If you were to use them as part of a larger “production” program, they would typically be combined into fewer files and minified by removing white space and comments. It is not recommended that you minify your Javascript code until you have a completely debugged implementation of your program. And you are highly encouraged to keep a non-minified version of your program around for future debugging and enhancements.
To learn how to develop WebGL programs, you will start with a simple working example and modify small parts of the system at one time. However, as we introduce advanced graphics concepts many parts of the software will have to change at the same time. This is one reason why we are starting these tutorials with the big picture in mind. You need to learn how small changes in one part of the software propagates through the other parts.
Since you will be studying code examples and modifying existing code, we need to discuss coding standards in the next section.
Glossary¶
- JavaScript
- the programming language supported by web browsers that allows for the modification of web pages. JavaScript runs on the client computer and performs client side processing.
- object oriented programming
- a style of programming where a class defines a collection of data and a set of methods/functions that manipulates that data. Multiple instances of a class can be created to implement separate objects of the class.
- class
- a collection of data and a set of methods/functions that manipulates that data.
- object
- an instance of a class that contains unique data. The manipulation of an object does not affect other objects implemented from the same class.
- global identifier
- a variable or function that can be used anywhere in a program. Global identifiers are bad and should be avoided whenever possible.