9.8 - Types of Light Sources¶
In lesson 9.1 we discussed various types of light sources. Let’s repeat the list here for review.
- Point light source: The light is inside the scene at a specific location and it shines light equally in all directions. An example is be a table lamp. Point light sources are modeled using a single location, (x,y,z,1).
- Sun light source: The light is outside the scene and far enough away that all rays of light are basically from the same direction. An example is the sun in an outdoor scene. Sun light sources are modeled as a single vector, <dx, dy, dz, 0>, which defines the direction of the light rays.
- Spotlight light source: The light is focused and forms a cone-shaped envelop as it projects out from the light source. An example is a spotlight in a theatre. Spotlights are modeled as a location, (x,y,z,1), a direction, <dx.dy,dz,0>, a cone angle, and an exponent that defines the density of light inside the spotlight cone.
- Area light source: The light source comes from a rectangular area and projects light from one side of the rectangle. An example is a florescent light fixture in a ceiling panel. Area light sources are modeled as a rectangle, (4 vertices), and a direction, <dx.dy,dz,0>.
All of the lighting calculations we have discussed in lessons 9.2-9.5 used a point light source. This lesson discusses the modifications needed to implement other types of light sources.
Sun Light Source¶
The location of a sun light source is far away from a scene. If you calculated a vector from a vertex in the scene to the light source position you would get the same vector every time (assuming limited precision floating point math). Therefore, the location of the sun light source is modeled as a single vector that points towards the sun. Your JavaScript program should normalize the vector which eliminates the need for the fragment shader to normalize it – which makes the fragment shader execute faster. Your JavaScript code must also transform the sun light direction to “camera space” since lighting calculations are being done in “camera space.” The light direction vector is copied to a shader program as a uniform variable before rendering begins.
Using the sun’s light direction as a “vector to the light source”, all calculations for ambient, diffuse, and specular light remain unchanged.
A sun light source is so far away from a scene that it is basically the same distance away from all vertices in the scene. Therefore you would typically not implement light attenuation.
Spotlight Light Source¶
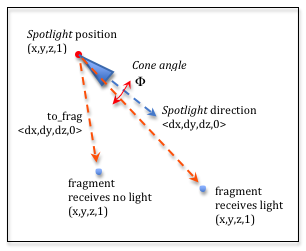
Spotlight light source.
A spotlight light source has a position in the scene, but its light is restricted to a particular direction. To determine if light from a spotlight is reaching a fragment you can perform the following calculations:
- Calculate a vector from the spotlight location to the fragment. (Let’s call this vector to_frag.)
- Calculate the angle between the to_frag vector and the direction vector of the spotlight.
- If the angle is less than or equal to the spotlight’s cone angle, then the fragment is receiving light from the spotlight. If the angle is bigger than the spotlight’s cone angle, the fragment is not receiving any light from the spotlight.
Once you have determined that a fragment is receiving light, you can calculate the diffuse and specular light as we previously discussed. If the fragment is not receiving light from the spotlight, the only coloring the fragment receives is from ambient light.
Be careful with the direction of the vectors you calculate and make sure they are consistent with each other. For example, if you calculate a vector from a fragment to the spotlight, this vector will be going in the opposite direction from the spotlight direction and you will calculate the wrong angle.
The intensity of the light within the spot light is not typically uniform. The intensity is greatest at the center of the cone and least at the edges. You can use a cosine function raised to a power as we did for specular reflection to model the varying intensity of light inside the cone.
Area Light Source¶
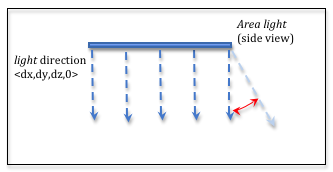
Area light source.
An area light source models the typical lighting in the ceiling of an office. Light comes from a rectangular area and projects down into a room. The major difference between this type of light source and a point light source is that the location of the light is not unique. There are multiple ways that you can model an area light. Here is one way:
- Determine if the fragment is on the side of the area light that is receiving light. If it is not, use only ambient light. Otherwise, continue.
- Project a fragment’s location onto the plane that contains the area light rectangle.
- Determine if the projected point is inside the rectangle.
- If the projected point is inside the rectangle, use this location as the location of the light source.
- If the projected point is outside the rectangle, use the closest point on the border of the rectangle as the light source.
Once you have a light source location, and you can perform the diffuse and specular calculations.
You can model the light as projecting in a single direction, or as spreading out from the boundaries based on a specified angle. You can make the calculations as simple or as complex as you want. Typically the more complex the model, the better the visual effects.
Multiple Light Sources in a Scene¶
A typical scene has multiple light sources. For such cases you perform the calculations we have previously described for each light source and then simply add the resulting colors. Light is additive!
If you have light sources that are being turned on and off at various times during a scene animation, you have two basic choices:
- Include a uniform boolean variable in your shader program that enables or disable the lighting calculations for a particular light source.
- Define multiple shader programs and use the appropriate shader program for each lighting situation.
Using if statements in shader programs slows down their execution speed. If rendering speed is an issue, you should use a separate shader program for each possible lighting situation. If rendering speed is not an issue, you should use a single shader program with boolean flags to control lighting calculations.
Glossary¶
- point light source
- A light source that is inside a scene. The light source projects light in all directions.
- sun light source
- A light source that is external to a scene. All light rays from the light source have the same direction.
- spotlight light source
- A light source that is inside a scene, but its light rays are restricted to a single direction within a cone-shaped envelope.
- area light source
- A light source that projects light from a rectangular area in a single direction.