10.8 - Transformations on Texture Maps¶
Texture coordinates are often defined for a model and never modified. This allows for the surface properties of a model to remain static, even while the geometry of a model is being transformed in location, orientation, and scale. But there are times when you would like a texture to move across the surface of a face, such as a surface that represents water in an ocean. The water is constantly moving due to wind and other forces. So how can we move a texture map? It’s simple, we move the texture coordinates that defines its location.
This lesson introduces the basic ideas behind texture map transformations.
Overview¶
Transformations on 2D texture coordinates are basically the same transformations applied to 3D geometry. The only difference is that we are dealing with flat surfaces that have only 2 dimensions, which means we use a 3-by-3 transformation matrix (instead of a 4-by-4 transformation matrix). The following table lists the standard transformations and their associated matrices.
Transformation | Transform Matrix |
---|---|
Translate | 1.0 0.0 0.0 0.0 1.0 0.0 tx ty 1.0 |
Rotate | cos(angle) sin(angle) 0.0 -sin(angle) cos(angle) 0.0 0.0 0.0 1.0 |
Scale | sx 0.0 0.0 0.0 sy 0.0 0.0 0.0 1.0 |
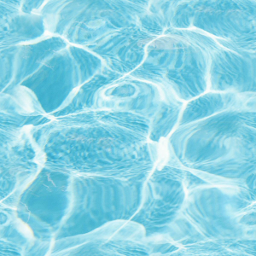
A “tileable” texture image (1)
We need a JavaScript library that can create 3-by-3 transformation matrices and perform math operations on them. The file Learn_webgl_matrix3.js in the demonstration program below contains the needed functionality. (Use the Chrome “development tools” to view or save a copy.)
In the GLSL language a mat3 data type holds a 3-by-3 matrix transformation. All matrices in WebGL are stored as 1D arrays organized in column-major order.
If we are going to move a texture map around by changing its texture coordinates, it is preferred that we use a “tileable” image that can be repeated over a surface. We set the texture map mode to gl.REPEAT so that texture coordinates that are transformed outside the range 0.0 to 1.0 map to a valid location in the texture image. The 256x256 image to the right is a “tileable” image.
Experimentation¶
As you experiment with the demonstration program below, please note the following:
- The texture coordinates for a model are stored in a buffer object in the GPU’s memory and that data never changes. Each time the model is rendered, a texture map transformation matrix is set to a uniform variable in the shader program. Each texture coordinate of the model is multiplied by this 3-by-3 transform in the vertex shader. The data is static; what is changing is the transformation.
- In the vertex shader, when you multiply a (s,t) texture coordinate times a 3-by-3 transformation matrix you must change the (s,t) value to (s,t,1). The additional component of 1 is the “homogeneous coordinate” for 2D coordinates. Remember that the 1 in the third position implements the translation part of the transformation.
- For a cube it is not possible to align the six sides of the cube in a way that does not produce visible “seams.” However, for more complex models you can typically get all the edges on one side of the model to align and then “hide” any seams that do not align on the back or bottom side of the model.
An example of transforming texture coordinates to make a texture map "move."
Translate texture: (0.00, 0.00) | Scale texture: (1.0,1.0) | Rotate texture: 0.0 degrees |
TX: -2.0 +2.0 | SX: -5.0 +5.0 | Angle: -180.0 +180.0 |
TY: -2.0 +2.0 | SY: -5.0 +5.0 |
Summary¶
Moving a texture map across the surface of a face is straightforward and uses the same transformation tools we have studied previously. In addition, the transformation is performed in the vertex shader so the impact on rendering speed is very minimal.
Glossary¶
- texture map transformation
- Change the location of texture coordinates at render-time using a 3-by-3 transformation matrix that contains translation, rotation, and scaling.
- tileable texture map image
- An image that can be placed in tiles over a plane and the seams between individual tiles is not discernible.
- homogeneous coordinate
- An additional component to a vector that extends the dimension of the vector. For computer graphics, a homogeneous coordinate allows translation to be included in a transformation matrix.